Customers come to your website looking for information and of course information changes. So it’s natural for site owners to want to be able to maintain their own sites, saving the delay and cost of having a designer make minor changes. Visitors also tend to respond favourably to a website which is “alive” and kept up to date. “Will I be able to update the site myself?” is a very common question to ask.
The answer generally is yes and there are a few steps involved in making that happen.
Developing a website on a Content Management System (CMS) makes updates easy for non-technical users. A CMS provides site owners with a private administration area they can log into to control site content. Implementing a CMS is a very common requirement, but it does add a little work to the process compared to a ‘static’ site, which is just a collection of HTML files. Supporting a CMS means that the design you and your designer come up with needs to be coded as a theme which ties the front end templates (HTML and CSS) to the CMS features.
Content Management System Options
There are a lot of options out there, but a few CMS are occupying the lions share of the market now. Some common choices are:
- WordPress
- A Custom Built Solution
- Concrete5
- Joomla
- Drupal
There are also ecommerce focused systems such as WooCommerce (an extension of WordPress), Lemon Stand and Magento.
WordPress has been the fastest growing and mostly widely used option for a few years now and is my go-to option for most new sites. It’s easy to use and has a critical mass of usage and development that makes it hard to ignore. WordPress has grown out of its origins as a blogging system and now supports pages and custom content types (e.g. products, services, offices, etc.).
There’s a rich eco-system of plugins, themes and developers for WordPress and it has a very well implemented updates system.
A custom built solution may also be appropriate in some cases, but they would be the minority. One advantage of a custom system would be a very easy learning curve for the site owners as there’d be no extraneous features in the result.
Knowing your Content
For some websites the site structure will be a series of simple pages that owners can edit through a basic editor (a text area similar to Microsoft Word or Google Docs) in the site’s backend. Often times though your business will have a little more structure to your content and the CMS can be improved with a little development work to better match the nature of your information.
For example if your business had offices in six locations, each with a phone number, primary email and street address (with corresponding map) you could simply type that information into a standard page. The solution would work, but it’d be difficult for those maintaining the site to keep the formatting consistent. Structuring the phone numbers and maps in the correct way would be tedious. A better approach would be to create what’s called a “Custom Post Type” for Offices during the site’s development. Users would then have dedicated form fields for those pieces of information and the templates created by the designer would be responsible for maintaining a consistent design. This approach reduces the work involved in keeping the site up to date, and makes the office data available for other users (e.g. listing all offices in a footer or sidebar automatically).
Here’s how that might look to site editors in WordPress:
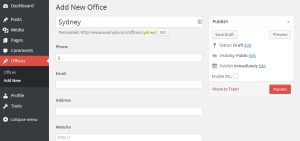
Training
The training requirements for staff to use a system like WordPress are pretty minimal; usually under an hour. It’s often a matter of resizing images to a sensible scale before uploading and knowing where to find the screens you need.
The extra time taken to develop your site on top of a CMS like WordPress or even programming a simple custom system for your business can be worthwhile. Site owners usually enjoy the flexibility of being able to make changes to their own site whenever they want without having to email and pay their designer to do it. The ease of maintenance can also mean more frequent updates which only helps you to get more value out of your website.