AWS Cloudfront is a CDN for delivering content to your users faster, by serving it from locations closer to them. It caches requests nearer to users and call pull the original content from its S3 service, or your own web server.
By default a Cloudfront distribution comes with an SSL enabled subdomain on the cloudfront.net domain name that looks something like this:
dlksg932809.cloudfront.net //default example
cdn.mikehealy.com.au //custom domain
You can also create a free SSL certificate through AWS for your own custom domain (or subdomain) and point that to your Cloudfront distribution. This is especially useful if you’re using Cloudfront to serve a static site in lieu of a conventional server.
Creating a Free, Custom SSL through AWS Certificate Manager
Go to AWS’ Certificate Manager product and choose ‘Request a certificate’.
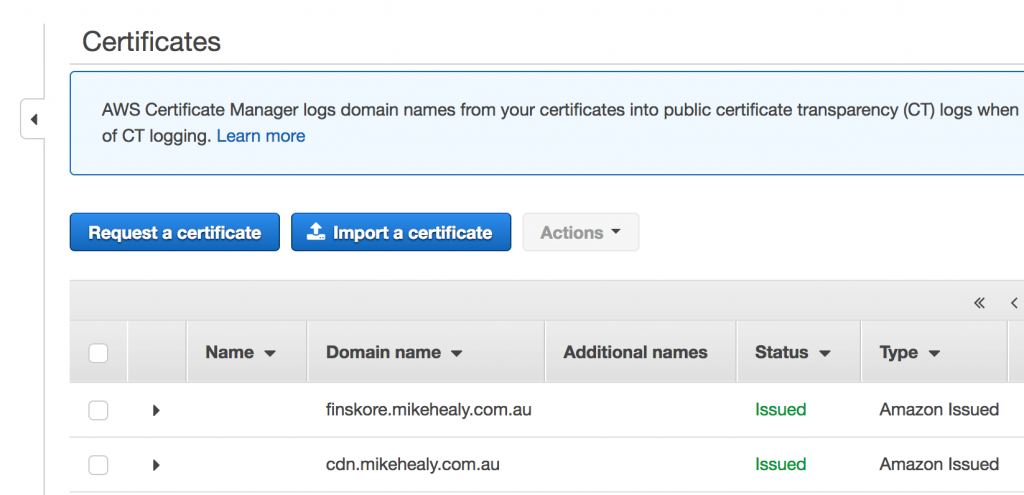
You’ll be asked to specify your domain (or subdomain) and which validation method to use.
DNS Validation
This method has you setting a CNAME record for the domain to prove that you have control of the domain. It’s the preferred method, however some DNS providers don’t support the characters required due to faulty validation rules, and so it might not be available to you.
Email Validation
This is the alternative method and requires you to have access to receive mail at a common admin email address such as administrator@example.com for your root domain.
The process after either creating your DNS CNAME or selecting email validation is essentially to follow the prompts and let AWS provision a new certificate for you.
Setting up your Cloudfront Distribution
Once your certificate has been created you can create your new distribution and select it as the SSL certificate to use. The other options for your distribution aren’t covered in this post, but you can now use your own (sub)domain to point to this distribution and your choice of S3 or your own web server to act as the origin server.
Provisioning the distribution takes a little while, usually more than 15 minutes in my experience.
Once that’s happened you’ll have a great, low cost static file serving distribution on your own domain with free SSL!
This is great for low-cost side projects or serving static files for your main website that would not otherwise justify setting up a web server and configuring Lets Encrypt, or having to purchase a traditional SSL certificate.
CDNs for WordPress
You can use a plugin (of course) to rewrite your content URLs so they are served off your CDN. This leaves the editing and publishing process unchanged. The plugin will handle converting local URLs to CDN versions.
As mentioned you have the choice of using your local web server, or S3 as the origin for your Cloudfront distribution. One advantage of moving your media off your WordPress site and onto S3 as the main store is that your local site install stays smaller and is therefore easier to move and backup.
Useful WordPress / S3 / CDN plugins